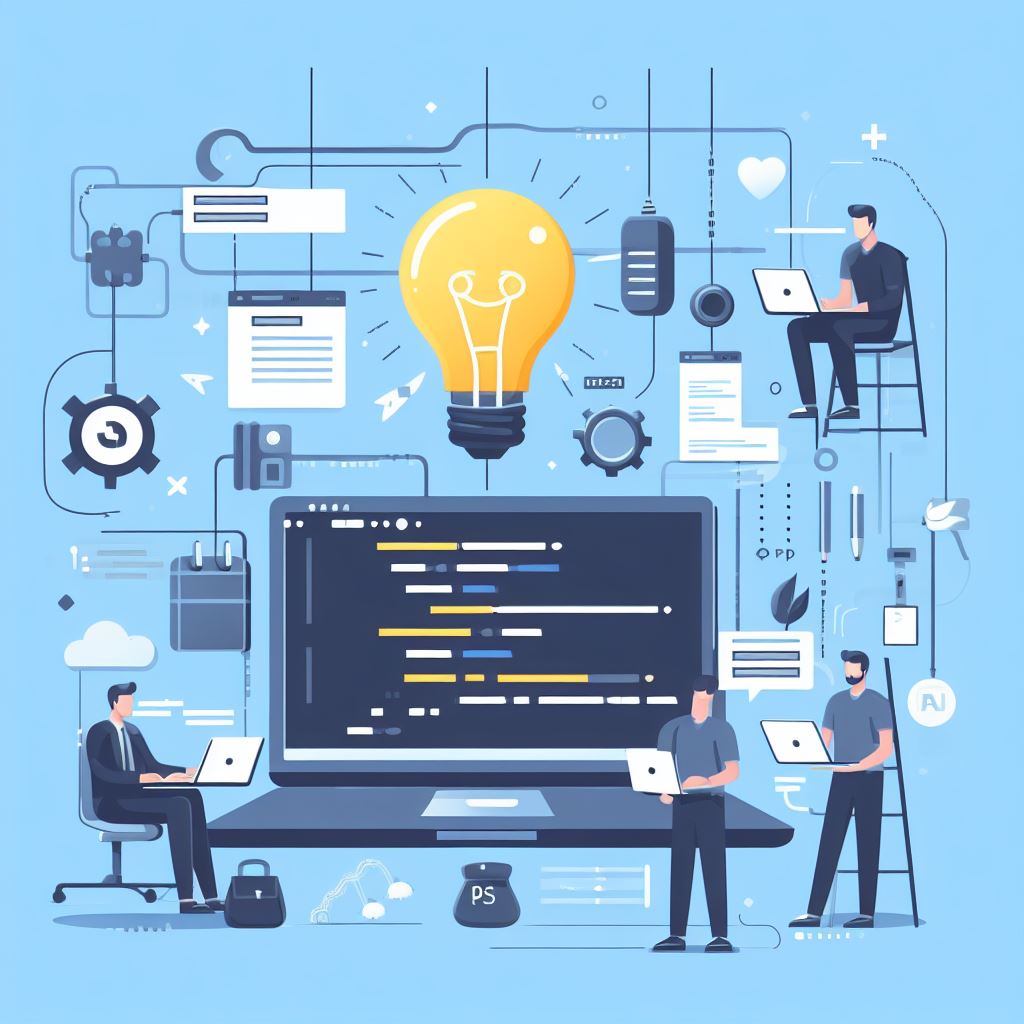
PHP to find the file size in bytes
PHP to find the file size
PHP to find the file size in bytes
In PHP, you can use the filesize
function to get the size of a file in bytes. Here’s an example:
$filename = 'path/to/your/file';
$filesize = filesize($filename);
echo "The size of the file is $filesize bytes.";
This code will output the size of the file in bytes. Please replace 'path/to/your/file'
with the actual path to your file.
Please note that the result of the filesize
function is cached. If you want to clear the cache, you can use the clearstatcache
function.
Also, be aware that because PHP’s integer type is signed and many platforms use 32-bit integers, some filesystem functions may return unexpected results for files which are larger than 2GB.
PHP find file size in kilo bytes and mega bytes
In PHP, you can use the filesize
function to get the size of a file in bytes.
To convert this size into kilobytes (KB) or megabytes (MB), you can divide the number of bytes by 1024 for KB, or by 1024 twice for MB.
Here’s an example of how you can do this:
$filename = 'path/to/your/file';
// Get file size in bytes
$filesize_in_bytes = filesize($filename);
// Convert file size to kilobytes (KB) and megabytes (MB)
$filesize_in_kb = $filesize_in_bytes / 1024;
$filesize_in_mb = $filesize_in_kb / 1024;
echo "The size of the file is $filesize_in_bytes bytes, $filesize_in_kb KB, or $filesize_in_mb MB.";
This code will output the size of the file in bytes, kilobytes, and megabytes. Please replace 'path/to/your/file'
with the actual path to your file.
Please note that the result of the filesize
function is cached. If you want to clear the cache, you can use the clearstatcache
function.
Also, be aware that because PHP’s integer type is signed and many platforms use 32-bit integers, some filesystem functions may return unexpected results for files which are larger than 2GB.
How to take kb upto two decimal places?
To round the file size to two decimal places in kilobytes (KB), you can use the round
function in PHP. Here’s how you can do it:
$filename = 'path/to/your/file';
// Get file size in bytes
$filesize_in_bytes = filesize($filename);
// Convert file size to kilobytes (KB) and round to 2 decimal places
$filesize_in_kb = round($filesize_in_bytes / 1024, 2);
echo "The size of the file is $filesize_in_kb KB.";
This code will output the size of the file in kilobytes, rounded to two decimal places. Please replace 'path/to/your/file'
with the actual path to your file.
Please note that the result of the filesize
function is cached. If you want to clear the cache, you can use the clearstatcache
function.
Also, be aware that because PHP’s integer type is signed and many platforms use 32-bit integers, some filesystem functions may return unexpected results for files which are larger than 2GB.
This content was generated by Microsoft Bing. (source)