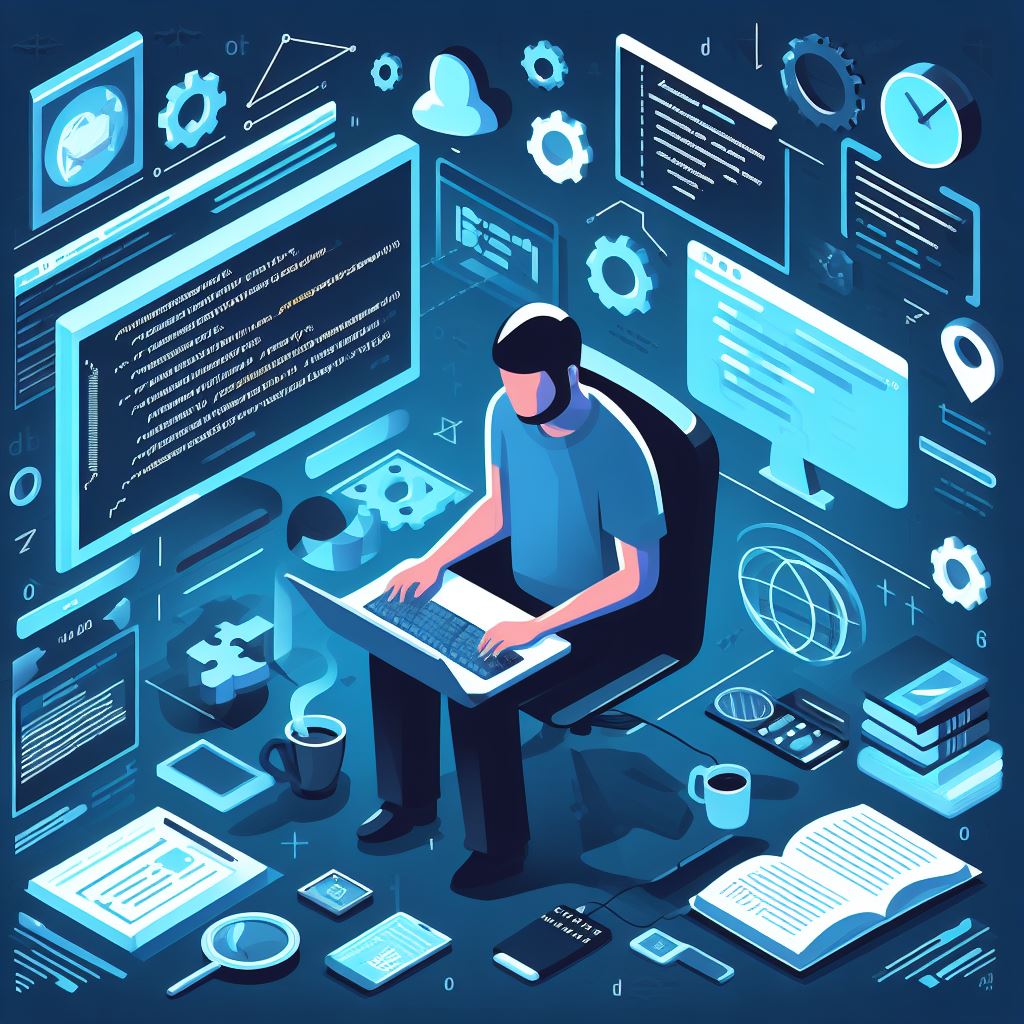
PHP sort by name and date on button click
PHP sort by name and date on button click
To sort a list by name and date upon clicking a button or an anchor tag in PHP, you can use the $_GET
superglobal to check if a sort request has been made and then adjust your SQL query accordingly.
Here’s an example:
<?php
// Connect to your database
$db = new PDO('mysql:host=localhost;dbname=testdb;charset=utf8', 'username', 'password');
// Set default order
$order = 'name';
$sort = 'asc';
// Check if sort order is set
if (isset($_GET['order']) && isset($_GET['sort'])) {
$order = $_GET['order'];
$sort = $_GET['sort'] == 'asc' ? 'desc' : 'asc';
}
// Get data from database
$stmt = $db->prepare("SELECT * FROM your_table ORDER BY $order $sort");
$stmt->execute();
$results = $stmt->fetchAll(PDO::FETCH_ASSOC);
?>
<!-- Display data -->
<table>
<tr>
<th><a href="?order=name&sort=<?php echo $sort; ?>">Name</a></th>
<th><a href="?order=date&sort=<?php echo $sort; ?>">Date</a></th>
</tr>
<?php foreach ($results as $row): ?>
<tr>
<td><?php echo $row['name']; ?></td>
<td><?php echo $row['date']; ?></td>
</tr>
<?php endforeach; ?>
</table>
In this code, clicking on the ‘Name’ or ‘Date’ headers will sort the table by that column. The sort order will toggle between ascending and descending each time the header is clicked.
This content was generated by Microsoft Bing. (source)
Was this helpful?
How was this post?
Subscribe to our newsletter
Stay updated on new releases and features, guides, and case studies.
Get tips, technical guides, and best practices. Once a month. Right
in your inbox.